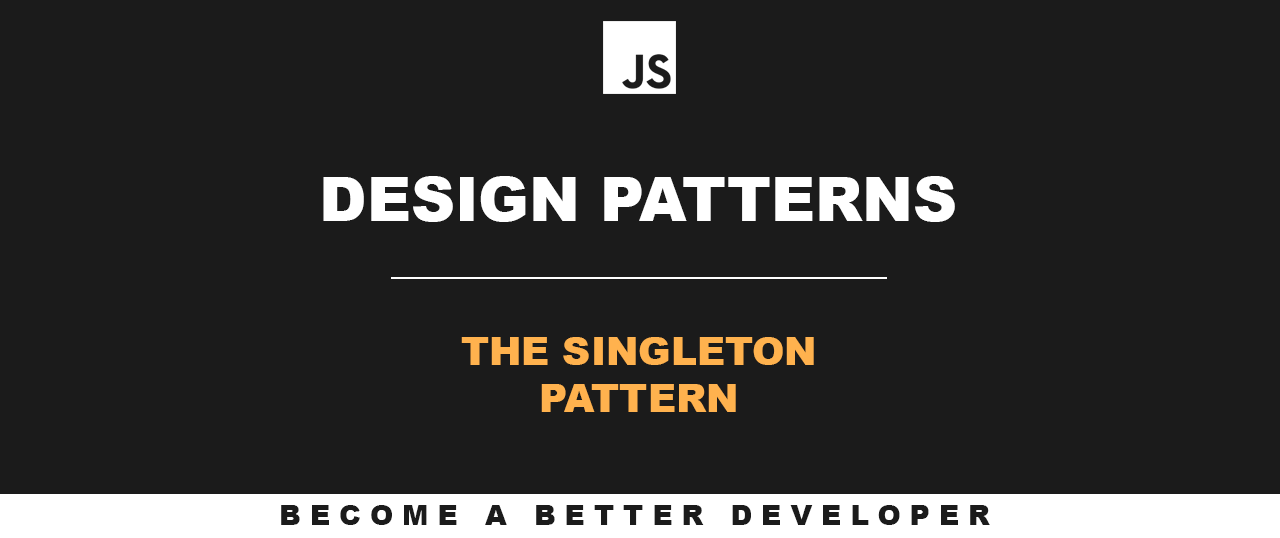
Unlocking JavaScript Design Patterns: Mastering Singleton for Ultimate Code Efficiency
Oct 23 2023
In the world of JavaScript, design patterns are your secret weapon to crafting efficient, organized, and maintainable code. Among these patterns, the Singleton pattern stands out as a versatile powerhouse, enabling you to ensure a single instance of a class throughout your application. Let's see how it works !
The Singleton Pattern Demystified
The Singleton pattern, at its core, guarantees that a class has only one instance and provides a global point of access to that instance. This means that no matter how many times you request an instance of the class, you will always get the same one. Singleton is particularly useful when you need to manage shared resources or control access to a single point, such as a configuration manager, database connection, or, in our case, a logging system.
The Logger Example
Setting Up the Logger
Imagine you're building a JavaScript application that consists of multiple modules and files. Debugging and tracking issues across the application can become challenging without a unified logging system. This is where the Singleton pattern comes to the rescue.
Let's create a simple logger that will maintain a single log throughout the application. Here's our logger.js
file:
In this implementation, we've defined a Logger
class that allows logging messages and displaying them. The getInstance
static method ensures that only one instance of the Logger
class exists throughout your application.
Use the Singleton Logger
Now, let's see how we can utilize the Singleton logger in different parts of our application.
app.js (Usage in One File)
In app.js
, we import the Logger instance, create a loggerInstance1
, and use it to log messages. The beauty of the Singleton pattern is that we are always working with the same instance, ensuring that all logs are stored in one centralized location.
anotherFile.js (Usage in Another File)
In anotherFile.js
, we again import the same Logger
instance and use loggerInstance2
. Despite being in a different file, loggerInstance2 is essentially the same instance as loggerInstance1
. This means that all logs from both files are accumulated in the same log array, giving you a comprehensive overview of your application's behavior.
Benefits of the Singleton Logger
Implementing the Singleton pattern for a logger in your JavaScript application offers several benefits:
1 - Centralized Logging: All log messages are collected in one place, making it easier to trace application behavior and debug issues.
2 - Consistency: You can be confident that you're working with the same logger instance throughout your application, ensuring data integrity.
3 - Easy Integration: The Singleton logger seamlessly integrates into different parts of your code, enhancing overall code efficiency.
Conclusion
The Singleton pattern is a powerful tool in JavaScript, providing a straightforward way to manage shared resources and maintain a single point of control in your application. As demonstrated through our logger example, it ensures that you have one consistent logger instance, simplifying debugging and enhancing code efficiency.
By mastering the Singleton pattern and using it wisely, you can unlock the full potential of your JavaScript applications, making them more organized, maintainable, and efficient.