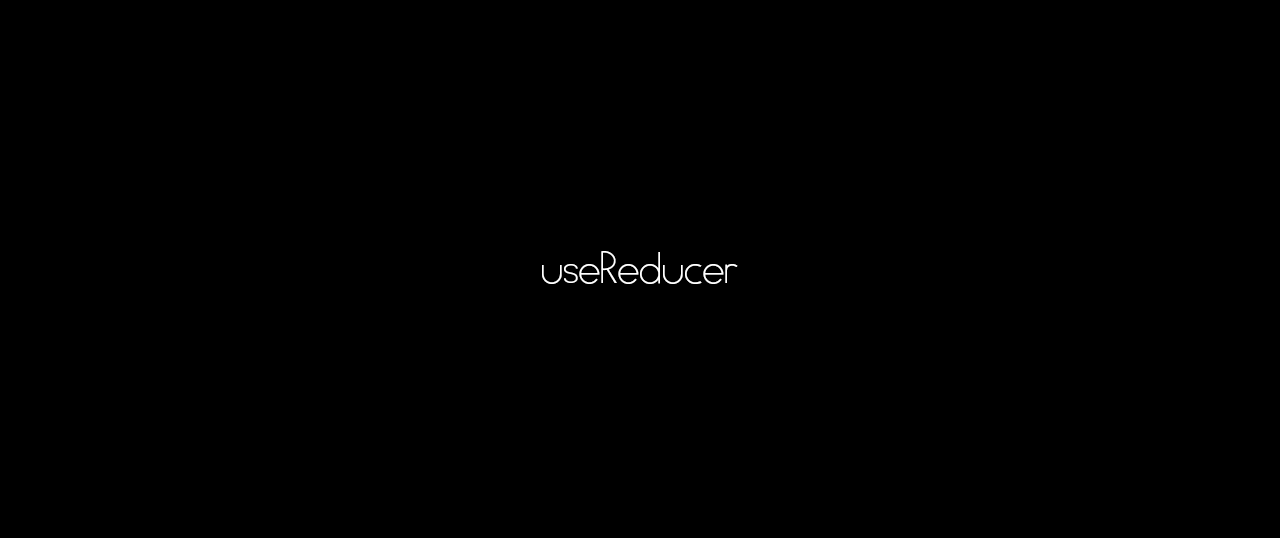
Revolutionize Your React App with useReducer: Mastering State Management
Nov 13 2023
State management is at the core of any React application, and for more complex scenarios, the useReducer
hook provides a powerful way to manage state in a structured manner. In this article, we'll explore useReducer through a concrete example: creating a to-do list.
Understanding useReducer
useReducer
is a React hook that allows state management using a reducer pattern. Instead of using useState
to manage a component's state, useReducer
is particularly suitable for handling complex states, such as lists of data.
The basic idea is to provide a reducer function that takes two arguments: the current state and the action to perform.
- The action is typically an object that describes a change in state, such as adding a task, deleting it, or marking it as done.
- The reducer function returns the new state based on the current state and the action.
Pratical exemple : Creating a To-Do List with useReducer
Imagine you're developing a to-do list application. Here's how you can use useReducer
to manage the list of tasks:
In this example, we've created a to-do list application. We use useReducer
to manage the tasks. Actions like ADD_TASK
, DELETE_TASK
, and TOGGLE_TASK
allow us to modify the state of the task list in a structured way.
Benefits of useReducer
for Task Management
useReducer
brings several advantages for managing a to-do list in a React application:
-
Structure and Readability: Actions are explicitly defined with types, making the code more readable and maintainable.
-
Immutable State: useReducer encourages state immutability, which avoids side effects and unexpected errors.
-
Handling Complex States: useReducer is ideal for managing a list of data with many possible actions.
-
Separation of Concerns: By grouping actions and reduction logic in a single function, useReducer promotes the separation of concerns.
-
Potential for Future Extensions: You can easily add new actions to extend your application's functionality.
Conclusion
In conclusion, useReducer
is a powerful tool for managing the state of a React application, especially when dealing with complex states and a variety of possible actions.
By incorporating it into your projects, you can create more robust and structured applications while improving code maintainability and readability.